Synchronous & Asynchronous Reset
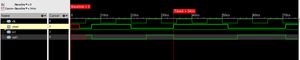
Reset
Reset is a signal that is used to initialize the hardware, as the design does not have a way to do self initialization. That means, reset forces the design to a known state. In simulation, usually it is activated at the beginning, but in real hardware, reset is usually activated to power up the circuits.
There are two types of resets used in hardware designs. They are synchronous and asynchronous resets.
Synchronous reset
Synchronous reset means reset is sampled with respect to clock. In other words, when reset is enabled, it will not be effective till the next active clock edge.
module synchronous_reset_test (input logic clk, reset, in1, output logic out1) always @(posedge clk) if(reset) out1 <= 1'b0; else out1 <= in1; endmodule
In the above example, you can see that out1 will be changed only with the posedge of clk. To get the effect of reset, reset should be wide enough to be captured by the next posedge of clk.
Advantages: 1. Gives a completely synchronous circuit 2. Provides filtering for the reset signal, So circuit will not be affected by glitches. (Special case: If glitch happens at the active clock edge, reset signal will be affected.) 3. Will meet reset recovery time, as the deassertion will happen within 1 clock cycle
Disadvantages 1. Reset needs to be stretched, if it is not long enough to be seen at the active clock edge. 2. Requires presence of clock to reset the circuit. 3. Asynchronous reset may be required if there are internal tri state buffers. 4. It is slow. 5. Synthesis will not be able to easily differentiate reset from other signals. So this has to be taken care while doing synthesis. Otherwise it may lead to timing issues. 6. If there are gated clocks for power saving, this type of reset wont be suitable.
Asynchronous reset
In asynchronous reset, reset is sampled independent of clk. That means, when reset is enabled it will be effective immediately and will not check or wait for the clock edges.
module asynchronous_reset_test (input logic clk, reset, in1, output logic out1); always @(posedge clk or posedge reset) if(reset) out1 <= 1'b0; else out1 <= in1; endmodule
Advantages 1. Reset gets the highest priority. 2. It is fast. 3. Does not require presence of clock to reset the circuit.
Disadvantages 1. Reset line is sensitive to glitches. 2. May have metastability issues
Comparison
module test; logic clk,reset,in1; wire out1;
//uncomment below code & comment async while simulating synchronous design //synchronous_reset_test srst(clk,reset,in1,out1); asynchronous_reset_test asrst(clk,reset,in1,out1);
initial clk <= 1'b0; always #5 clk = !clk;
initial begin in1 <= 1'b1; #3 reset <= 1'b0; #4 reset <= 1'b1; #13 reset <= 1'b0; #14 reset <= 1'b1; #18 reset <= 1'b0; #19 reset <= 1'b1; end
initial #75 $finish; endmodule
module synchronous_reset_test (input logic clk, reset, in1, output logic out1) always @(posedge clk) if(reset) out1 <= 1'b0; else out1 <= in1; endmodule
module asynchronous_reset_test (input logic clk, reset, in1, output logic out1); always @(posedge clk or posedge reset) if(reset) out1 <= 1'b0; else out1 <= in1; endmodule
Waveforms from the simulation (using above code) for synchronous and asynchronous circuits are shown below.
Synchronous Design
Asynchronous Design
In this we can see that, synchronous changes are happening with respect to clock. In asynchronous whenever there is a posedge of clock or posedge of reset out1 will be changed.
Conclusion
Both synchronous and asynchronous reset have advantages and disadvantages. These would be used as per the design needs. For example if chip has to be powered up prior to clock, asynchronous reset has to be used. Similarly if you want to design a completely synchronous circuit with no metastability issue related to reset, go with synchronous reset.